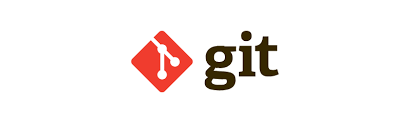
This blog post gives you a good overview of GitHub and also lists down the commands i keep handy for my day to day work. This article will also help you in your Devops/Git related interview questions
Table of Contents
What is Github?
used for Source control management (SCM).
Following are the few things you need to know:
1. cloning – pulling down the copy of the source code from the remote repo
2. adding – once changes are made to the source code, it needs to be added to be committed.
3. committing – adds the change to source control repository
4. pushing – pushing changes from local repo to global repo
5. branching – separate group of changes are kept in separate branch, e.g. new branch for feature development.
6. merging – merge the branch with master branch
7. pull requests – implemented by github, to allow review before merge.
Installing & configuring Git
sudo yum -y install git
git –version -> to verify installation
Once installed, set the name and email associated with your git commits.
git config –global user.name “<user name>”
git config –global user.email <user email>
Once configured, you need to set up private key access. Use ssh key pair to authenticate with remote git server.
generate a key using keygen command:
ssh-keygen -t rsa -b 4096
copy the contents of: ~/.ssh/id_rsa.pub
go to github.com and paste the content at settings->ssh and gpg keys section.
click new ssh key and enter the name and the content copied into the key field.
Basics of Git File System
When you create a local repo, a folder .git is added to that directory
when you add a file to the directory, you need to use “git add” so as to let git know that git needs to track this file.
git add puts the file in the staging area within the directory. – > virtual location -> git uses to track changes to file.
within your directory, you will have lines of development, known as branches.
when you commit the added file, it gets stored in the branch.
Git Repository listing:
commit_editmsg – file – each commit to the repo is recorded here.
config – config information related to developer
description – name of repo
hooks- scripts that you create to enable some custom action – automated
index – files in staging area
logs – logs
Useful Git Commands
to create a git repository:
git init “directory”
git init –bare “directory”
initializes a bare git repository, for larger projects, contains no working area
used when you have many contributors.
git config
command used to configure various elements of your git environment
added to track who made changes to the file
git config –global user.name “kunal”
git config –global user.email “kunal@gmail.com”
view your configuration:
git config –list
you can even configure the above two config per repo as well as global. git config will pick up the last value stored in its config file. It stores them as key value pair.
git add “filename.txt”
– now tracked by git, in the staging area
git status
– to see what files are in the staging area
git does not care of directories, only files.
touch src/.keep -> another way to add directory to git staging area
add keep to staging area
git rm
-remove the file from git
git rm -f
– forcefully remove
git status -s
– less verbose
A – added
M- modified – if you modify the file which is just added. when you commit, the M part will not be committed.
git status -v
– verbose output
git commit
– open the commits in text pad, type in the message there
all commit messages are recorded in commit_editmsg file
git commit -m “message”
git rm –cached “filename”
-removes the committed file from git, but keeps it in the local working directory
git commit -a -m “filename”
-adds the file modified in the staging area
git – ignoring files -> .git/info/exclude
.gitignore file -> used to ignore patterns of file local to git repo
git add .gitignore
git commit -m .gitignore
git check-ignore <pattern>
– shows what is ignored by git, based on your pattern
tag
acts like a stocky note. Often times, marked with version number for a release
annotated tag
git tag -a <tag-name> -m message
-marked for release or referenced in automation
contains full objects and database of your commits
lightweight tag
git tag <tag-name> -m <message>
is meant for lightweight label
Branch
git branch <branch-name>
master is default
git checkout <branch name>
switch to another branch
to see commits in just one line
git log –oneline –decorate
git merge
combines the latest commits from two branches in one branch
git branch -d branch_name
delete the specified branch
git rebase <branch>
-replay changes made to one branch over the top of another branch.
It simply copies over the changes
reverting a commit:
git revert <commit>
-revert a commit in project
git revert head -> revert the previous commit
git log –oneline
git revert head~2 for going two level down from head
or simply copy the commit id to revert
git diff
view the differences between two commits, files, blobs or between the working tree and staging area
git diff –summary commit1 commit2
git gc
cleans out old objects that can not be references by the db anymore and compresses the content within .git directory to save disk space
git gc –prune
cleans out the object that are older than two weeks
git gc –auto
to check if repo needs cleaning
Git log
git log –oneline
git clone <local-repo> <new-repo>
– to avoid messing up prod copy
cloning remote repositories
git clone <repo-url>
the remote project will be downloaded to our system
forking – as simple as making a copy of that repo
git pull git fetch
fetches new commit information down from the remote server for the current repo, does not commit anything to local db.
-> tells if you are up-to-date with the remote repo and merges as well
-> must do fetch or pull before committing changes to remote repo.
git push -u <remote> local
pushes local changes to remote repository
pull request
Now that you have your contributions to a project pushed to the remote repository, you will need to create a pull request to get it incorporated